Merge branch 'master' of https://github.com/JustinChia/variant-form
commit
70bb4b0c16
|
@ -0,0 +1,252 @@
|
|||
# Variant Form iView版
|
||||
#### 一款高效的Vue低代码表单,可视化设计,一键生成源码,享受更多摸鱼时间。
|
||||
|
||||
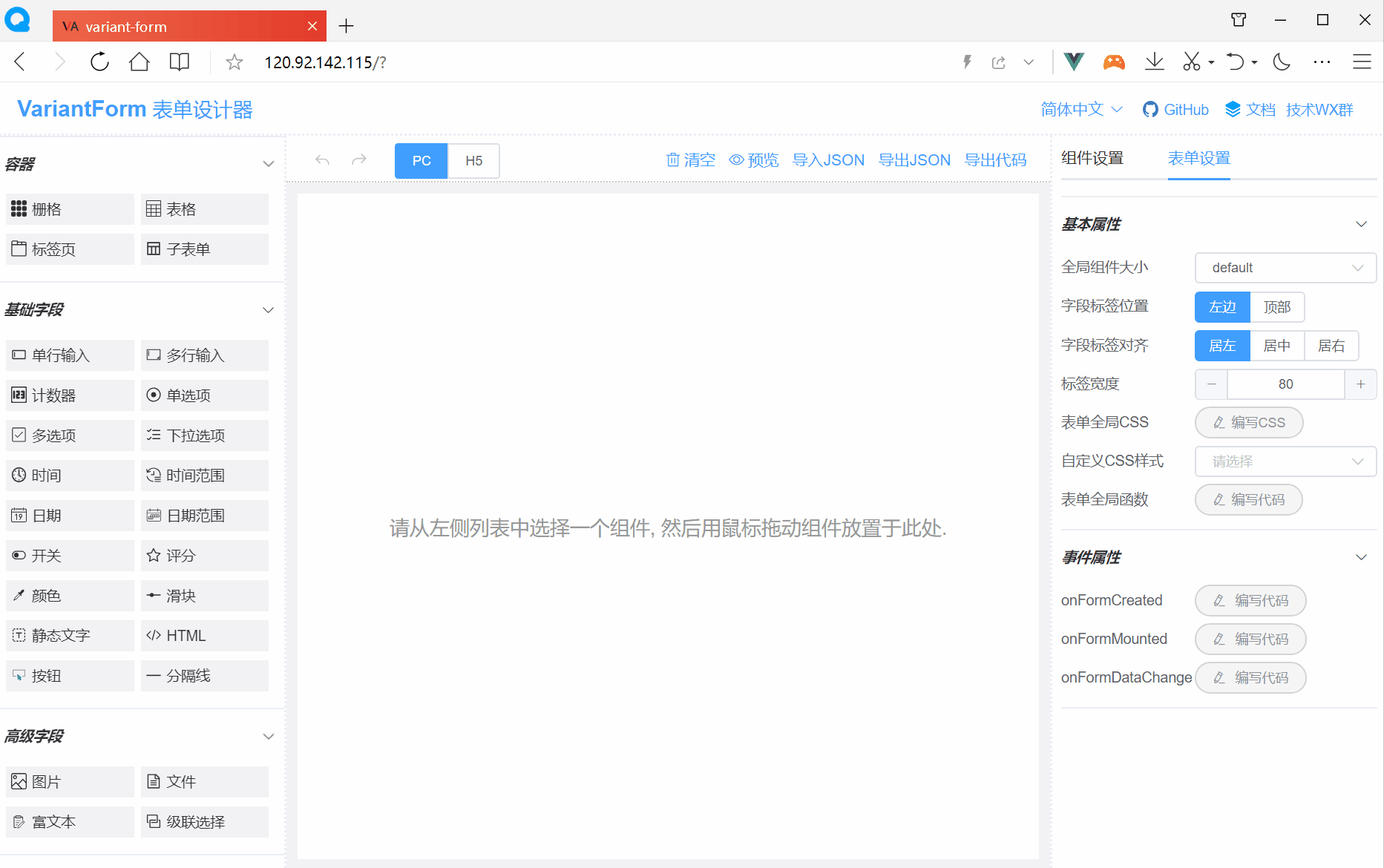
|
||||
|
||||
<br/>
|
||||
|
||||
### 安装依赖
|
||||
```
|
||||
npm install
|
||||
```
|
||||
|
||||
### 开发调试
|
||||
```
|
||||
npm run serve
|
||||
```
|
||||
|
||||
### 生产打包
|
||||
```
|
||||
npm run build
|
||||
```
|
||||
|
||||
### 表单设计器 + 表单渲染器打包
|
||||
```
|
||||
npm run lib-iview
|
||||
```
|
||||
|
||||
### 表单渲染器打包
|
||||
```
|
||||
npm run lib-render-iview
|
||||
```
|
||||
|
||||
### 浏览器兼容性
|
||||
```Chrome(及同内核的浏览器如QQ浏览器、360浏览器等等),Firefox,Safari,IE 11```
|
||||
|
||||
<br/>
|
||||
|
||||
### 跟Vue项目集成
|
||||
|
||||
|
||||
|
||||
###### 打包
|
||||
|
||||
```
|
||||
//VFromDesigner:
|
||||
npm run lib-iview
|
||||
//VFromRender
|
||||
npm run lib-render-iview
|
||||
```
|
||||
|
||||
####### 使用vFormDesigner的lib包
|
||||
1. main.js全局注册
|
||||
```
|
||||
...
|
||||
import ViewUI from 'view-design';
|
||||
import VFormDesigner from './{YOURPATH}/VFormDesigner.umd.min.js'
|
||||
import './{YOURPATH}/VFormDesigner.css'
|
||||
...
|
||||
Vue.use(VFormDesigner);
|
||||
Vue.use(ViewUI, {size:'small'});
|
||||
...
|
||||
new Vue({
|
||||
render: h => h(App)
|
||||
}).$mount('#app')
|
||||
```
|
||||
2. 在Vue模板中使用组件
|
||||
```
|
||||
<template>
|
||||
<v-form-designer></v-form-designer>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
export default {
|
||||
data() {
|
||||
return {
|
||||
}
|
||||
}
|
||||
}
|
||||
</script>
|
||||
|
||||
<style lang="scss">
|
||||
body {
|
||||
margin: 0; /* 如果页面出现垂直滚动条,则加入此行CSS以消除之 */
|
||||
}
|
||||
|
||||
|
||||
```
|
||||
|
||||
|
||||
####### 使用vFormRender的lib包
|
||||
1. main.js全局注册
|
||||
```
|
||||
...
|
||||
import ViewUI from 'view-design';
|
||||
import VFormRender from './{YOURPATH}/VFormRender.umd.min.js'
|
||||
import './{YOURPATH}/VFormRender.css'
|
||||
...
|
||||
Vue.use(VFormRender);
|
||||
Vue.use(ViewUI, {size:'small'});
|
||||
...
|
||||
new Vue({
|
||||
render: h => h(App)
|
||||
}).$mount('#app')
|
||||
```
|
||||
2. 在Vue模板中使用组件
|
||||
```
|
||||
<template>
|
||||
<div>
|
||||
<v-form-render :form-json="formJson" :form-data="formData" :option-data="optionData" ref="vFormRef">
|
||||
</v-form-render>
|
||||
<el-button type="primary" @click="submitForm">Submit</el-button>
|
||||
</div>
|
||||
</template>
|
||||
<script>
|
||||
export default {
|
||||
data() {
|
||||
return {
|
||||
formJson: {"widgetList":[],"formConfig":{"labelWidth":80,"labelPosition":"left","size":"","labelAlign":"label-left-align","cssCode":"","customClass":"","functions":"","layoutType":"PC","onFormCreated":"","onFormMounted":"","onFormDataChange":""}},
|
||||
formData: {},
|
||||
optionData: {}
|
||||
}
|
||||
},
|
||||
methods: {
|
||||
submitForm() {
|
||||
this.$refs.vFormRef.getFormData().then(formData => {
|
||||
// Form Validation OK
|
||||
alert( JSON.stringify(formData) )
|
||||
}).catch(error => {
|
||||
// Form Validation failed
|
||||
this.$message.error(error)
|
||||
})
|
||||
}
|
||||
}
|
||||
}
|
||||
</script>
|
||||
```
|
||||
|
||||
|
||||
|
||||
<br/>
|
||||
|
||||
#### 1. 安装包
|
||||
```bash
|
||||
npm i vform-builds
|
||||
```
|
||||
或
|
||||
```bash
|
||||
yarn add vform-builds
|
||||
```
|
||||
|
||||
<br/>
|
||||
|
||||
#### 2. 引入并全局注册VForm组件
|
||||
```javascript
|
||||
import Vue from 'vue'
|
||||
import App from './App.vue'
|
||||
|
||||
import ViewUI from 'view-design' //引入iView库
|
||||
import VForm from 'vform-builds/dist/VFormDesigner-iView.umd.min.js' //引入iView版本VForm库文件
|
||||
|
||||
import 'view-design/dist/styles/iview.css' //引入iView样式
|
||||
import 'vform-builds/dist/VFormDesigner-iView.css' //引入VForm样式
|
||||
|
||||
Vue.config.productionTip = false
|
||||
|
||||
Vue.use(ViewUI, {size:'small'}) //全局注册iView
|
||||
Vue.use(VForm) //全局注册VForm(同时注册了v-form-designer和v-form-render组件)
|
||||
|
||||
new Vue({
|
||||
render: h => h(App),
|
||||
}).$mount('#app')
|
||||
```
|
||||
|
||||
<br/>
|
||||
|
||||
#### 3. 在Vue模板中使用表单设计器组件
|
||||
```html
|
||||
<template>
|
||||
<v-form-designer></v-form-designer>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
export default {
|
||||
data() {
|
||||
return {
|
||||
}
|
||||
}
|
||||
}
|
||||
</script>
|
||||
|
||||
<style lang="scss">
|
||||
body {
|
||||
margin: 0; /* 如果页面出现垂直滚动条,则加入此行CSS以消除之 */
|
||||
}
|
||||
</style>
|
||||
```
|
||||
|
||||
<br/>
|
||||
|
||||
#### 4. 在Vue模板中使用表单渲染器组件
|
||||
```html
|
||||
<template>
|
||||
<div>
|
||||
<v-form-render :form-json="formJson" :form-data="formData" :option-data="optionData" ref="vFormRef">
|
||||
</v-form-render>
|
||||
<el-button type="primary" @click="submitForm">Submit</el-button>
|
||||
</div>
|
||||
</template>
|
||||
<script>
|
||||
export default {
|
||||
data() {
|
||||
return {
|
||||
formJson: {"widgetList":[],"formConfig":{"labelWidth":80,"labelPosition":"left","size":"","labelAlign":"label-left-align","cssCode":"","customClass":"","functions":"","layoutType":"PC","onFormCreated":"","onFormMounted":"","onFormDataChange":""}},
|
||||
formData: {},
|
||||
optionData: {}
|
||||
}
|
||||
},
|
||||
methods: {
|
||||
submitForm() {
|
||||
this.$refs.vFormRef.getFormData().then(formData => {
|
||||
// Form Validation OK
|
||||
alert( JSON.stringify(formData) )
|
||||
}).catch(error => {
|
||||
// Form Validation failed
|
||||
this.$message.error(error)
|
||||
})
|
||||
}
|
||||
}
|
||||
}
|
||||
</script>
|
||||
```
|
||||
|
||||
<br/>
|
||||
|
||||
### 资源链接
|
||||
<hr>
|
||||
|
||||
文档官网:<a href="http://www.vform666.com/" target="_blank">http://www.vform666.com/</a>
|
||||
|
||||
在线演示:<a href="http://demo.vform666.com/" target="_blank">http://demo.vform666.com/</a>
|
||||
|
||||
VS Code插件:<a href="http://www.vform666.com/pages/plugin/" target="_blank">http://www.vform666.com/pages/plugin/</a>
|
||||
|
||||
Github仓库:<a href="https://github.com/vform666/variant-form" target="_blank">https://github.com/vform666/variant-form</a>
|
||||
|
||||
Gitee备份仓库:<a href="https://gitee.com/vdpadmin/variant-form" target="_blank">https://gitee.com/vdpadmin/variant-form</a>
|
||||
|
||||
更新日志:<a href="http://www.vform666.com/pages/changelog/" target="_blank">http://www.vform666.com/pages/changelog/</a>
|
||||
|
||||
技术交流群:微信搜索“vformAdmin”,或者扫如下二维码加群
|
||||
|
||||
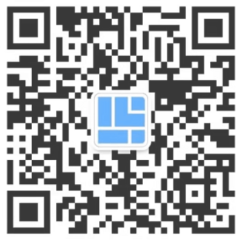
|
|
@ -543,51 +543,7 @@
|
|||
v-if="hasConfig('labelTooltip')">
|
||||
<Input type="text" v-model="optionModel.labelTooltip" />
|
||||
</FormItem>
|
||||
|
||||
<!-- 复合输入框 -->
|
||||
<FormItem :label-width="0" v-if="hasConfig('appendControl')">
|
||||
<Divider size="small" class="custom-divider">{{i18nt('designer.setting.inputControl')}}
|
||||
</Divider>
|
||||
<div style="font-size:12px;line-height:15px;color:#AAAAAA">提示:受iview组件限制,切换前置/后置组件可能无法及时生效,请刷新页面</div>
|
||||
</FormItem>
|
||||
<!-- 添加前置按钮 -->
|
||||
<FormItem :label="i18nt('designer.setting.prependControl')" v-if="hasConfig('prependControl')">
|
||||
<Checkbox v-model="optionModel.prependControl" @on-change="updateFormView"></Checkbox>
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.prependControlDisabled')" v-if="hasConfig('prependControlDisabled')">
|
||||
<Checkbox v-model="optionModel.prependControlDisabled"></Checkbox>
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.prependControlIcon')" v-if="hasConfig('prependControlIcon')">
|
||||
<Input type="text" v-model="optionModel.prependControlIcon" />
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.prependControlType')" v-if="hasConfig('prependControlType')">
|
||||
<Select v-model="optionModel.prependControlType">
|
||||
<Option v-for="item in inputControlType" :key="item.value"
|
||||
:label="item.label" :value="item.value">
|
||||
</Option>
|
||||
</Select>
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.prependControlText')" v-if="hasConfig('prependControlText')">
|
||||
<Input type="text" v-model="optionModel.prependControlText" />
|
||||
</FormItem>
|
||||
<!-- 添加后置按钮 -->
|
||||
<FormItem :label="i18nt('designer.setting.appendControl')" v-if="hasConfig('appendControl')">
|
||||
<Checkbox v-model="optionModel.appendControl" @on-change="updateFormView"></Checkbox>
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.appendControlDisabled')" v-if="hasConfig('appendControlDisabled')">
|
||||
<Checkbox v-model="optionModel.appendControlDisabled"></Checkbox>
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.appendControlIcon')" v-if="hasConfig('appendControlIcon')">
|
||||
<Input type="text" v-model="optionModel.appendControlIcon" />
|
||||
</FormItem>
|
||||
<FormItem :label="i18nt('designer.setting.appendControlType')" v-if="hasConfig('appendControlType')">
|
||||
<Select v-model="optionModel.appendControlType">
|
||||
<Option v-for="item in inputControlType" :key="item.value"
|
||||
:label="item.label" :value="item.value">
|
||||
</Option>
|
||||
</Select>
|
||||
</FormItem>
|
||||
|
||||
|
||||
<FormItem :label="i18nt('designer.setting.appendControlText')" v-if="hasConfig('appendControlText')">
|
||||
<Input type="text" v-model="optionModel.appendControlText" />
|
||||
</FormItem>
|
||||
|
|
|
@ -240,20 +240,13 @@ export default {
|
|||
showWordLimit: 'Show Word Limit',
|
||||
prefixIcon: 'Prefix Icon',
|
||||
suffixIcon: 'Suffix Icon',
|
||||
inputControl: 'Complex Input Setting',
|
||||
prependControl: 'Prepend Control',
|
||||
prependControlDisabled: 'Disable Prepend Control',
|
||||
prependControlType: 'Prepend Control Type',
|
||||
prependControlText: 'Prepend Control Type',
|
||||
appendControl: 'Append Control',
|
||||
appendControlDisabled: 'Disable Append Control',
|
||||
appendControlType: 'Append Control Type',
|
||||
appendControlText: 'Append Control Text',
|
||||
buttonIcon: 'Button Icon',
|
||||
activeText: 'Active Text',
|
||||
inactiveText: 'Inactive Text',
|
||||
activeColor: 'Active Color',
|
||||
inactiveColor: 'Inactive Color',
|
||||
inputButton: 'Input Button Setting',
|
||||
appendButton: 'Append Button',
|
||||
appendButtonDisabled: 'Button Disabled',
|
||||
buttonIcon: 'Button Icon',
|
||||
switchWidth: 'Width of Switch(px)',
|
||||
activeText: 'Active Text',
|
||||
inactiveText: 'Inactive Text',
|
||||
maxStars: 'Stars Max Number',
|
||||
lowThreshold: 'Low Threshold',
|
||||
highThreshold: 'High Threshold',
|
||||
|
|
|
@ -239,21 +239,14 @@ export default {
|
|||
maxLength: '最大长度',
|
||||
showWordLimit: '显示字数统计',
|
||||
prefixIcon: '头部Icon',
|
||||
suffixIcon: '尾部Icon',
|
||||
inputControl: '复合输入框设置',
|
||||
prependControl: '添加前置按钮',
|
||||
prependControlDisabled: '前置按钮禁用',
|
||||
prependControlType: '前置按钮类型',
|
||||
prependControlIcon: '前置按钮图标',
|
||||
prependControlText: '前置按钮文字',
|
||||
appendControl: '添加后置按钮',
|
||||
appendControlDisabled: '后置按钮禁用',
|
||||
appendControlType: '后置按钮类型',
|
||||
appendControlIcon: '后置按钮图标',
|
||||
appendControlText: '后置按钮文字',
|
||||
buttonIcon: '后置按钮Icon',
|
||||
activeText: '开启时文字描述',
|
||||
inactiveText: '关闭时文字描述',
|
||||
suffixIcon: '尾部Icon',
|
||||
inputButton: '输入框按钮设置',
|
||||
appendButton: '添加后置按钮',
|
||||
appendButtonDisabled: '后置按钮禁用',
|
||||
buttonIcon: '后置按钮Icon',
|
||||
switchWidth: '开关宽度(像素)',
|
||||
activeText: '开启时文字描述',
|
||||
inactiveText: '关闭时文字描述',
|
||||
activeColor: '开启时背景色',
|
||||
inactiveColor: '关闭时背景色',
|
||||
maxStars: '最大评分值',
|
||||
|
|
Loading…
Reference in New Issue